BLACKSheep® Coding Guideline: Unterschied zwischen den Versionen
en>Peter K (1 Version importiert) |
Peter (Diskussion | Beiträge) K (1 Version importiert) |
(kein Unterschied)
|
Aktuelle Version vom 31. Oktober 2023, 09:03 Uhr
Scope
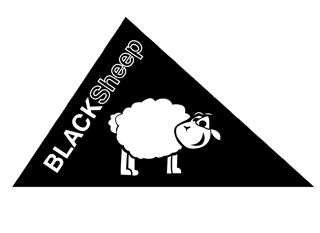
This page contains naming, structure and design rules for writing C code
at BLACKSheep® OS for Blackfin® processors.
Naming of identifiers
Every identifier has to be named in English.
The first character of an identifier must not contain underscores (there are some compiler directives which start with underscores and this could lead to conflicts)!
Variables
- Mixed case letters has to be used and the appropriate prefixes have to be inserted where necessary.
- Variable names have to be self explanatory. The names have to be provided with the appropriate prefixes and they have to start with an uppercase letter.
In case of combining prefixes, the use of ranges/arrays/pointer/enumerations/structures is at first, followed by basic data types or object prefixes. - The only exception are loop variables (thereby the use of i, j, k is allowed).
- Only one variable declaration per line is allowed
- Pointer operators at the declaration have to be located in front of the variable (not after the type identifier).
- If possible Initializations have to be done directly at the declaration.
- Prefixes for ranges/arrays/pointer/enumerations/structures
Prefix | Description |
---|---|
g_ |
For global varaibles. |
s_ |
For static variables. |
pa_ |
For function parameters. |
a |
For arrays. |
fn |
For function pointers. |
p |
For pointers. |
t |
For self definend datatypes e.g. enumerations, structures. |
h |
For handlers. |
- Prefixes for basic data types
Prefix | Description |
---|---|
c |
For characters (8Bit). |
b |
For boolean (1Bit). |
n |
For datatype: int (32Bit) and long (32Bit). |
f |
For all kind of floating point datatypes. |
s |
For short(16Bit). |
ll |
For long long (64Bit). |
u |
Additional prefix to indicate an unsigned variable. |
- Prefixes for basic global variables
For global variables the prefix g_
should be used, followed by the type prefix.
Example
<source lang="c">
bool g_bIsInitialized; void aFunction (int pa_nParameter) { int nNumber; int *pnNumber = &nNumber; char cKey; float fPi = 3.1415; int anNumbers[10]; unsigned char * pucPac; }
</source>
Datatypes
Defining your own datatypes is common and necessary to improve code readability.
The notation for self defined datatypes is: <source lang="c">T_TYPE_DESCRIPTION</source>
- Example
<source lang="c">
typedef unsigned long T_UART_HANDLE;
typedef struct _EXAMPLE_CFG { bool bDTParalell; unsigned long unIVG; unsigned long unPPIerrIVG; unsigned long unDMAerrIVG; } T_EXAMPLE_CFG;
</source>
Blocks
The left parenthesis of a block has to be in the same line as the starting construct.
The right parenthesis has to be in an own line.
The parenthesis must ALWAYS be used! Also in single for or if statements!
- Never type something like this
<source lang="c">
if (x == 1) y = 10;
</source> or this: <source lang="c">
if (x==1) y=10;
</source>
BLACKSheep® conform notation
- if /else statements
<source lang="c">
if (...) { ... } else if (...) { ... } else { ... }
</source>
- For statements
<source lang="c">
for (...) { ... }
</source>
- Switch/case statements
<source lang="c">
switch (nVariable) { case 0 : { ... break; } case 1 : { ... break; } default : { ... } }
</source>
Functions
Functions should start with a three or four letter identifier common for all functions in a c-file.
The letters should be in upper case.
The left parenthesis of the function has to be in the same line as the last parameter and the close bracket.
- Example
<source lang="c">
T_ERROR_CODE UARTwriteByte(T_UART_HANDLE pa_tHndl, unsigned char pa_ucValue) { ... }
</source> If the number of parameters is too long to fit in one line the function declaration should be formatted as follow: <source lang="c">
T_ERROR_CODE UARTsetMode ( unsigned long pa_unBaudrate, unsigned char pa_ucStopBits, unsigned char pa_ucParity ) { ... }
</source>
Error codes
If the function returns an error code the T_ERROR_CODE declaration should be used (declared in “driver/include/datatypes.h”).
A generic error type called ERR_GENERIC is already defined there.
The “driver/include” path must be added to the project options as a standard include path.
All error values should be derived from ERR_GENERIC.
- Example
<source lang="c">
#include <datatypes.h> #define UART_ERR_RX_BUFFER_FULL (ERR_GENERIC – 10)
</source>
Include guards
The use of include guards is recommended. If used, they have to look like this: <source lang="c">
#ifndef _SAMPLE_H_INCLUDED_ #define _SAMPLE_H_INCLUDED_ ... #endif
</source>
Include paths
Include paths has to be relative. Don’t use absolute paths!
Use slashes as directory separator. Don’t use backslash!
- Example
<source lang="c">
#include “../vdm/vdmconfig.h”
</source> Include files from standard include directories (VDSP++ as well as BLACKSheep) has to be declared as follow: <source lang="c">
#include <stdio.h>
</source> Standard include directories for BLACKSheep are:
- Driver/ include
- Blacksheep/include
Both directories should be added as standard include directories on all VDSP++ projects.
Macros
Macro should be in capital letters and self explaining.
Macros should start with a three or four byte identifier related to the c-file or the function block.
As separator use the underline character.
- Example
<source lang="c">
#define UART_PARITY_NONE 0
</source>
Indentation
The tabulator width has to be set to four.
Instead of tabulator characters spaces have to be inserted (usually there is an option for this in the IDE).
In VDSP++: Settings {{#if: Prefernces | Prefernces {{#if: Editor Tab |
Editor Tab {{#if: |
{{{4}}} {{#if: |
{{{5}}} {{#if: |
{{{6}}} {{#if: |
{{{7}}} {{#if: |
{{{8}}} {{#if: |
{{{9}}} {{#if: |
{{{10}}}| }}| }}| }}| }}| }}| }}| }}| }}| }}.
A new block has to be started at the same line as its initial statement.
Comments
A sufficient amount of comments have to be written.
There are never too many comments, whereas invalid comments are worse than none – thus invalid comments have to be removed from the source code.
Comments have to be written in English. It is allowed to indicate Single-line comments with // ahead of the command or in the same line right after the command. All other comments have to be located ahead of the command or block.
Generally comments have to be tagged with // or the doxygen style ///< not /*…*/ to allow the temporarily commenting out of code with /* … */!
Comments have to be meaningful, to describe to program and to be up to date.
All c- and h-files in addition must have doxygen compatible comments as described here.