BLACKSheep® Doxygen Guideline
Scope
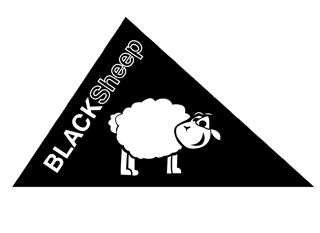
This page provides a short overview about the Doxygen Source Code documentation tool and how to add doxygen support to your source code.
Get more info about doxygen here.
You have to write your comments in a special style and the doxygen program parses your source code and can be configured in a very flexible way by a configuration file. Doxygen can generate a source code documentation as .rtf,.tex or as set of html pages.
Doxygen Syntax
Defining an Doxygen Block
Doxygen uses a slightly different syntax from standard c/c++ comment blocks.
Doxygen defines different comment styles which can be parsed by the program.
<source lang="c">
/** * line 1 * line 2 * line 3 **/ code ...
Code /// a single line Code ///< inline comment
</source>
Tags
In addition to simple comments doxygen features special keywords (Tags) in order to structure the output document .
These tags start with an “@” followed by the tag name.
- Important tags
Tag | Description |
---|---|
@file<name> |
Starts a documentation block for the file "name". |
@brief |
Brief description (one line). |
@author<name> |
Name of the authors. |
@version<name> |
Version of the source code. |
@pre<text> |
Description of preconditions. |
@post<text> |
Description of postconditions. |
@see<entity> |
Link to another doxygen entity. |
@param<name><description> |
Description of parameter of a function. |
@return<name><description> |
Description of the return value of a function. |
@attention<text> |
Add a warning text to a file and function. |
ingroup<name> |
Associate file to an group. |
defgroup <name><text> |
Define a group with name and description. |
What can/must be documented
- The following enties can be documented
- Files
- Functions
- Structs/enums
- Groups: entities can be grouped in doxygen . And these groups can be documented.
Entity | Property | Doxytag | required |
---|---|---|---|
file |
@file<name> |
Yes | |
Brief description | @brief<text> |
Yes | |
Author | @author<names> |
Yes | |
Version | @version<name> |
Optional | |
Date | @date |
Optional | |
Function |
|||
Brief description | @brief<text> |
Yes | |
Long description | Optional | ||
Parameter | @param<name><description> |
Yes | |
Return | @return<name><description> |
Yes | |
Non-trivial preconditions | @pre<text> |
Yes/Opt. | |
Non-trivial preconditions | @post<text> |
Yes/Opt. | |
Struct |
|||
Brief description | @brief<text> |
Yes | |
Long description | Optional |
Documenting an entity
File
Every file must contain a standard header featuring doxytags.
- Example
<source lang="c">
/** @file stringTools.h @ingroup String @brief Additional string functions This file contains helpful string functions which are not covered by <string.h> BLT_DISCLAIMER(TBD) @author Roland Oberhammer @version 1.0 @date 01.04.2010 **/
</source>
Function
Note that every parameter of the function and the return value are documented by using
@param tags- Documents in the function body should not be converted in doxytags.
<source lang="c">
/** @brief Split string by given seperator @param *pa_acSource source string @param pa_cSeparator Separator character @param **acSubstrings Output, pointer array to seperated substrings @return Number of substrings found **/ int strsplit(char *pa_acSource, char pa_cSeparator, char **acSubstrings) { register unsigned short nSubStrCount = 0; register unsigned short cIndex = 0; while (cIndex < strlen (pa_acSource)) { char *SubStr = acSubstrings[nSubStrCount]; while ((pa_acSource[cIndex] != pa_cSeparator) && (cIndex < strlen (pa_acSource))) { *SubStr = pa_acSource[cIndex]; SubStr ++; cIndex++; } *SubStr = 0; cIndex ++; nSubStrCount++; } return (int)nSubStrCount; }
</source>
Additional tags
In addition to the required parameters additional tags can be added if necessary.
Attention: @attention < text>
If a function has known bugs or restrictions which are not obvious .
A attention text should be added to notify the user about a special (You can also use @warning instead)
Postcondition: @post < text>
If this function enables some further steps after calling they could be noted here.
Precondition: @pre < text>
Steps that have to be taken before calling this function.
- Example
<source lang="c">
/** @brief Destructs your house @attention this function destroys your house! @param timer seconds to destruction @pre ApplyDynamite() called @post your house is destroyed **/ void Destruct_House(int pa_nSeconds ){ ... }
</source>
Struct
Structs/enums are documented with an generic header before the structure which features brief and long description.
Every member of the structure is described with an inline doxygen comment.
- Example
<source lang="c">
/** @brief device info structure to specify transfer parameters these parameters have different meanings for character devices (qualified with "on char-devices:") */ typedef struct { unsigned long nBlockCount; ///<number of blocks, on char-devices: receive buffer size unsigned long nBlockSize; ///<size of one block, on char-devices: undefined unsigned short nCylinderCount; ///<number of cylinders, on char-devices unsigned short nHeadCount; ///<number of heads, on char-devices unsigned short nBlocksPerTrack; ///<number of blocks per track, T_DEVICE_TYPE tDeviceType; ///<type of device (block device, character device) } T_DEVICE_INFO;
</source>
Defining Groups/Modules in Doxygen
Doxygen provides a mechanism to group several source files to modules and groups. A group must be defined once in the source code and then every file can be added to this group. Groups can even be added to other groups to create some sort of hierarchy.
A group can be defined by the following block: <source lang="c">@defgroup <name> (group title)</source>
- Example
<source lang="c">
/** @defgroup lowleveldrv_grp Low Level Driver contains low level hardware support @{@} */
</source>
Note that a group includes all entities between the @{ and the @} tags. But you can also add documented entities.
To add a file to specific group the file header must contain a tag “@ingroup groupname”
Creating a group hierarchy
You can also add groups to other groups to create a hierarchical structure of groups. <source lang="c">
/** @defgroup group3 The Third Group * This is the third group */ /** @defgroup group4 The Fourth Group * @ingroup group3 * Group 4 is a subgroup of group 3 */
</source>
@see tag
The @see tag can be used to link or point to another documented entities.
Syntax “@see <entity name>” <source lang="c">
/** @brief device info structure to specify transfer parameters these parameters have different meanings for character devices (qualified with "on char-devices:") @see CharacterDevicesGroup */
</source>
Bluetechnix Standard headers
File
<source lang="c">
/** * @file filename.c * @ingroup mygroup * * @brief Short description of the File/Module * * maybe a longer description * * which can go over several lines * * BLT_DISCLAIMER * * @author programmer1 , programmer2 * * @cond svn * * Information of last commit * $Rev:: $: Revision of last commit * $Author:: $: Author of last commit * $Date:: $: Date of last commit * * @endcond **/ /** * @defgroup mygroup My group * @ingroup othergroup * @brief short description * * Some text * * @{@} **/
</source>
Function
<source lang="c">
/** * @brief <text> * @attention <text> * * More text * * @param name <text> * @return <text> * @pre <text> * @post <text> **/
</source>
Struct/Enum
<source lang="c">
/** * @brief <text> * @attention <text> * * More text * * **/ typedef struct { type name; ///< Description } T_MY_STRUCT;
</source>
Defines and global variables
- Single line
<source lang="c">#define NAME VALUE ///< Description</source>
Create a group of defines (e.g. a group of error codes). <source lang="c">
/** * @name Groupname */ //@{ * #define NAME1 VALUE1 ///< text1 * #define NAME2 VALUE2 ///< text2 * #define NAME3 VALUE3 ///< text3 //@}
</source>